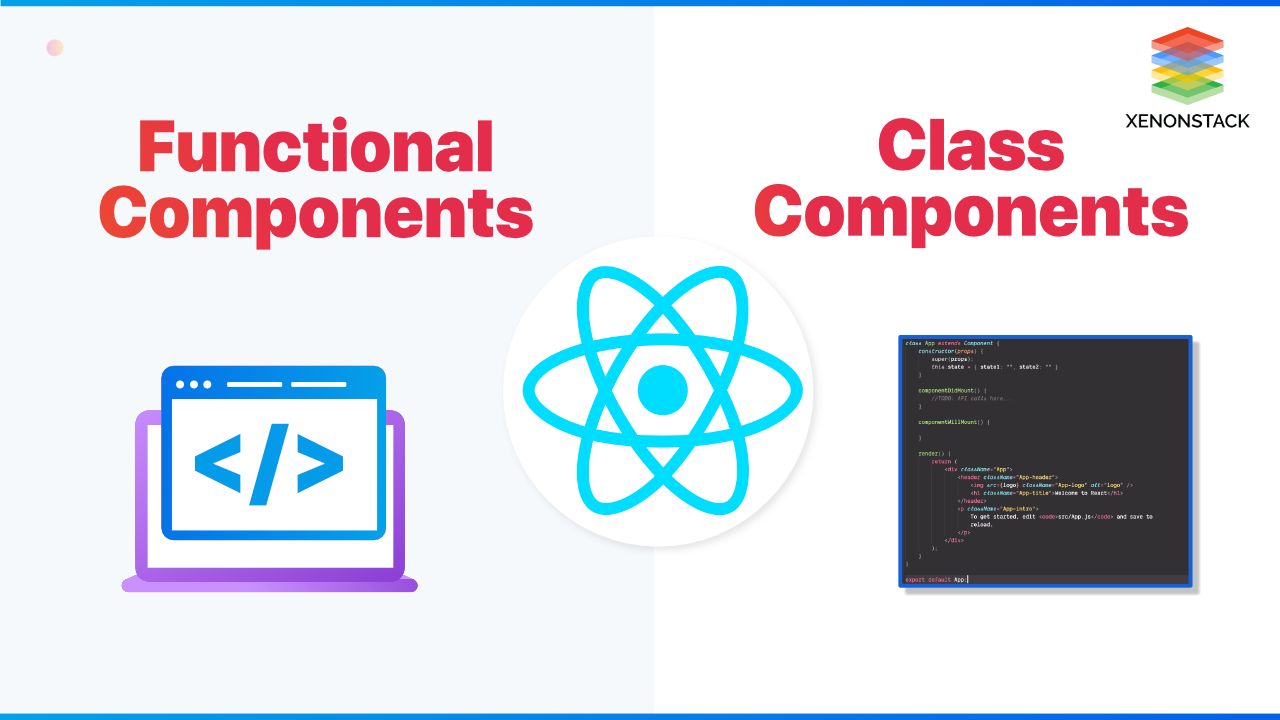
Introduction to Functional and Class-Components
In developing a single-page application, developers often write thousands of lines of code that follow the traditional DOM structure. To overcome this challenge, a component-based architecture is introduced, where the application is divided into React components.
A React component is considered a building block of the UI, making it easier to build. The final UI depends on which components are used, and they merge into a parent component.
React provides its primary functionality through functional components and class components, which are reusable components. These components are similar to JavaScript functions but return HTML and are isolated.
-
Faster Development Cycle
-
Reduced Cost
-
Improved Reliability
React functional components are simpler and follow a functional programming style, while class components use lifecycle methods. Stateful components can be created using React Hooks, like the useState hook and useEffect hook. Presentational components handle UI rendering, while container components manage logic and state.
A process of analyzing the performance of web applications with all their components. Click to explore about, How to Profile a React Application?
What Exactly Are React Functional and Class Components?
React components have their own structure, and over time, the developer community has added more features through libraries and tools for coding. React offers two types of components for creating UI elements: functional components and class components. Both components provide similar functionalities, and the choice depends on the developer’s preference. However, initially, class components were the only viable option for developing complex applications.
The reason behind this was that class components provided many usability features, such as lifecycle methods and state management. In contrast, functional components did not offer such options. However, with the release of React v16.8, an update introduced React Hooks, which allowed functional components to handle state management and lifecycle methods, making it possible to write complex applications using only functions as components.
Let’s explore the features available in the present day.
React server component works to re-write its components that are rendered on the server-side to enhance application performance. Click to explore about, Server Components Working
Functional Components
In reacting, they are just functions of Javascript functions like this:
function Sample(props) {
return hello world! Reading this blog? - {props.name};
}
const ele = <Sample name="Me!" />;
ReactDOM.render(ele, document.getElementById('home'));
In the above case, we render a user-defined component Sample. The element as ele passes JSX attribute name= ”ME” as a prop to the function Sample, which returns an < h1 > element as a result. For both types of components, props are inputs.
After importing it, we call this component like this :
import Sample from './sample';function App() {
return (
<div className="App">
<Sample />
</div>
);
}
So it must return JSX, Starts with Capital letter & follows pascal naming convention, takes props as a parameter if needed.
React 16 presented a new concept of an “error boundary” to solve a problem when a part in the UI contains a JavaScript error. Click to explore about, Error Handling in React 16
Class Components
There are additional features of these components, like maintaining logic and state. That's why it is called stateful or intelligent components.
-
You can use its lifecycle methods throughout the component like componentWillMount etc.
-
In components, we can access props pass down like this. props.
-
Class components make use of ECMAscript or ES6 class, then extend the component class.
class Checkyourscore extends React.Component {
render() {
return Hello! I'll calculate your marks!😎 {this.props.name};
}
}
It is an ES6 class that extends class components from its library. Here we used render() to return HTML.
Class components work fine with props. You can use syntax similar to an HTML attribute by using props to bypass a component. In the above example, we need to replace props.name with this.props.name in the render() body to use props. The state of a component has to initiate this.state that can be assigned with a class constructor.
Using React in application brings better performance and minimizes the number of DOM operations used to build faster user interfaces. Click to explore about, Optimizing React Application Performance
Key Differences Between Functional and Class Components Explained
The complete comparison is described below:
State and lifecycle
In this section, we will differentiate based on features as that class provides devs with such functionality as lifecycle and setState(). Methods like componentWillMount, componentDidUpdate, etc., while functional components don’t because these are simple JS functions that accept props and return their elements. In contrast, the class component has a render method that extends its Component. However, it had changed when Hooks came to the introduction. Component lifecycle classes have methods like componentDidUpdate. The component will unmount, shouldComponentUpdate. They have a tool that works the same as the mentioned methods using only one Hook, useEffect. So Hooks are like an addition for it rather than a replacement of class components.
Syntax
Different types of syntax are used in both components. Let’s look into some examples.
Declaring Components
- Functional components are JS functions:
function FunctionalComp() {
return <h1>Hello, sup world</h1>;
}
- Class components are classes that extends React.Component
class ClassComp extends React.Component {
render() {
return <h1>Hello, sup world</h1>;
}
}
Fot return <h1> tag we use render() method inside class component.
Passing Props
For example, there is a prop named: “Pickone”.
<component name = ‘First’ />
While using the functional component, we pass props as an argument of our function. “props.name” construction.
function FunctionalComp(props) {
return <h1>Hii, {props.name}</h1>;
}
While in-class class component, we add this. to refer props.
class ClassComp extends React.Component {
render() {
return <h1>Hii, {this.props.name}</h1>;
}
Handling State
For handling state in functional component React offer useState() Hook . let’s describe it using an example there is functionality added whenever we click it increases the count for this initial state of count set to 0 using method setCount() that increase the count every time click on the button.
const FunctionalCount = () => {
const [count, setCount] = React.useState(0);
return (
<div>
<p>count: {count}</p>
<button onClick={() => setCount(count + 1)}>Click this button</button>
</div>
);
};
We use the setState() function in the Class component, which requires a constructor and this keyword.
class ClassCount extends React.Component {
constructor(props) {
super(props);
this.state = {
count: 0
};
}
render() {
return (
<div>
<p>count: {this.state.count} per times clicked</p>
<button onClick={() => this.setState({ count: this.state.count + 1 })}>
Click this button
</button>
</div>
);
}
}
Here, both components have similar logic. State object, state key ‘count’ and initial value declared under constructor(). In render(), we use the function to update count value using this.state.count, and it renders the count of the button clicked also displays the button. Results are the same, but all the difference is that lines of code require more in-class components.
Hoisting only for functional components
According to the Hoisting concept, JS moves function and variable declaration to the top to access a variable or function, which allows access first, then declares it. While during the compile phase, it puts a declaration in memory that allows the calling function before declaring it. To get access to a class before the declaration throws a ReferenceError exception in classes.
Let’s look into an example where we call the function before its declaration:
petName(‘Ruby’);
function petName(name) {
console.log(‘My pet’s name is ’ + name);
}
// The result is: ‘My pet’s name is Ruby’
The above code will work fine, but it will get a reference error while declaring a class.
Like
const p = new MyName(); // ReferenceError
class MyName {}
Or we declare a variable and call it before the initialization returns undefined >
console.log(myName); // Returns undefined, as only declaration was hoisted
var myName; // Declaration
myName = “John”; // Initialization
Here, initialization with const and let are hoisted but not initialized, which means our app is aware of the existence of variables.
If we use a simple component that only returns a statement, there is no need to separate it and merge it into the root index.js file.
Performance difference
In this section, each developer might not consider this because it all depends on the usability of the developer. It shows a greater performance than class components. The point used to measure this is made of a simple object with the type(string) and props(object) 2 properties. Rendering such a component needs to call the function and passing props.
Class components are more complex because they are the instances of it. Component with constructor and complicated system of methods of using and manipulating state and lifecycle. In brief, theoretically, we can say calling a function takes less time than creating an instance of a class.
Understanding When to Use Functional or Class Components
Here's a quick comparison of functional components and class components, outlining when each type is best suited for use based on their features and functionality. The table below highlights the key differences between them:
When to Use |
Functional Components |
Class Components |
Simplicity & Conciseness |
Preferred for their simplicity, making them easier to write and understand |
Typically more verbose due to the need for class syntax |
Ideal for Stateless Components |
Best suited for creating stateless components or presentational components focused primarily on rendering UI |
Not ideal for stateless components, as they are designed to manage state |
Need for Lifecycle Methods |
No need for lifecycle methods. If your component doesn’t require managing state or handling lifecycle events, it's more efficient |
Required when needing explicit control over lifecycle methods like componentDidMount, componentDidUpdate, or componentWillUnmount |
State Management |
With React Hooks like useState and useEffect, functional components can manage state and handle side effects |
Necessary when you need to manage internal state directly before the introduction of hooks |
Complex Behavior |
Can handle most behaviors with React Hooks, though for more complex side effects, hooks may still be limited |
Better for complex behaviors and more controlled side effect handling, though functional components with hooks can handle most scenarios |
Key Considerations in Choosing Between Functional vs. Class Components
-
State Management: Use class components for internal state management before the introduction of hooks; functional components now support stateful components with useState hook.
-
Lifecycle Control: Class components are ideal when complex lifecycle methods are required, though React Hooks in functional components now provide similar control.
-
Performance: Functional components are generally more efficient and offer better performance, especially with modern optimizations like React.memo.
Advantages and Disadvantages of Functional and Class Components
Benefits of Using Functional Components
-
Simplicity: React functional components are easier to read and write due to their lightweight and declarative nature, making the codebase more maintainable.
-
No
this
Keyword: Functional components eliminate the need for thethis
keyword, reducing confusion, especially for developers new to JavaScript classes. -
Support for React Hooks: With React Hooks like useState hook and useEffect hook, functional components can manage state and handle side effects, offering much more power and flexibility without needing class components.
-
Performance Considerations: Functional components are generally faster because they are less complex, do not require the overhead of constructor methods, and do not rely on the render method like class components.
Challenges of Using Functional Components
-
Limited Features Before Hooks: Before the introduction of React Hooks, functional components were limited to being stateless components and couldn’t manage state or use lifecycle methods.
-
Complexity in Large Components: When functional components become large and incorporate complex logic or multiple hooks, they can become difficult to manage or maintain, especially in terms of readability and performance.
-
Limited Support for Advanced Lifecycle Events: While React Hooks have addressed most use cases, functional components might still face challenges in handling some advanced lifecycle behaviors in very complex scenarios, which class components handle more explicitly.
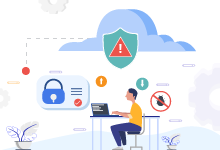
Benefits of Using Class Components
-
Lifecycle Methods: Class components provide built-in lifecycle methods (e.g.,
componentDidMount
,componentDidUpdate
,componentWillUnmount
) that give fine-grained control over the component's behavior, such as fetching data or handling side effects. -
State Management: Class components inherently support stateful components and make it easier to manage internal state without relying on React Hooks.
-
Explicit Structure: Class components have a more explicit structure with clearly defined methods, making them suitable for scenarios where the component’s lifecycle is complex and needs structured control.
Challenges of Using Class Components
-
Verbosity: Class components require more boilerplate code, such as the constructor method, render method, and
this
binding, making them more verbose compared to functional components. -
Learning Curve: For developers unfamiliar with JavaScript classes and the use of
this
in ES6 classes, class components can be challenging to understand and work with. -
Performance Overhead: Class components can introduce performance issues due to the additional overhead of constructor methods and render methods, especially when compared to the more lightweight functional components.
Best Practices for Using Functional and Class Components
- Modular Code and Functional Programming Style: Functional components align well with the functional programming style, promoting modularity and composition in a component-based architecture. Focus on creating small, reusable components that are easy to maintain and test, whether stateful or stateless.
- Performance Considerations: While functional components are typically faster, performance bottlenecks can arise with large or complex components, so using React.memo or memorization techniques can optimize performance. Minimizing unnecessary re-renders is key to maintaining efficient rendering in larger applications.
- Reusability: Functional components are ideal for creating reusable components because they are concise, declarative, and easier to maintain. Organize your components into container components to manage state and presentational components for rendering UI, ensuring reusability and separation of concerns.
Key Takeaways on React Component Types
The choice between functional components and class components depends largely on the complexity of the application. For less complex apps, developers may opt for functional components. However, with the introduction of React Hooks, this distinction has become less significant, as functional components can now handle state and lifecycle methods. Ultimately, the decision also depends on code readability, with functional components offering a more user-friendly and concise approach for most developers.
Next Steps for Implementing Functional and Class Components
Talk to our experts about implementing React functional components and class components, how different industries and departments use React components to enhance decision-making. Leverage React Hooks to automate and optimize operations, improving efficiency and responsiveness, similar to how stateful components and stateless components are managed in React.