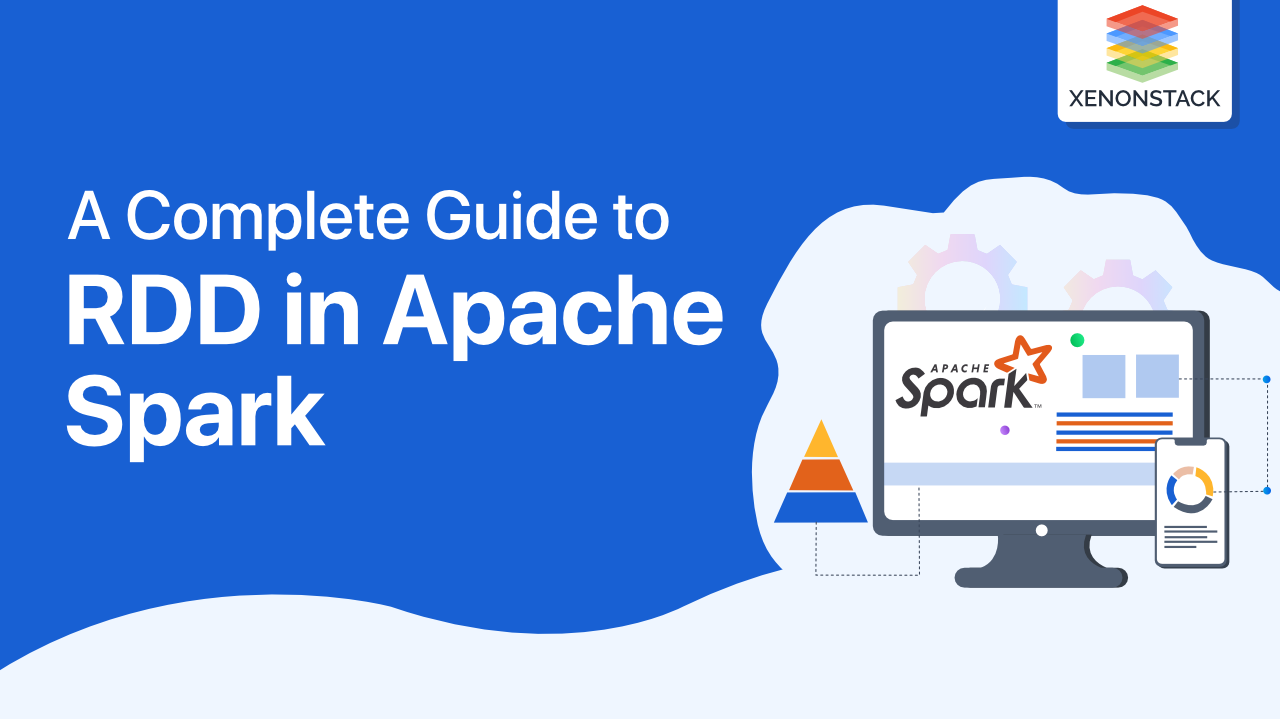
Understanding RDD: Key Concepts and Overview
Apache Spark is a powerful framework for big data processing, enabling in-memory computation and cluster computing at scale. At the core of its architecture is the Resilient Distributed Dataset (RDD), a fundamental abstraction for handling distributed data. RDD in Spark allows for efficient low-level transformations and actions on RDDs, making it ideal for unstructured data.
The RDD process is designed for fault tolerance, leveraging Spark Executors, the Spark Driver, and the Cluster Manager. Unlike DataFrames in Spark, RDDs offer schema-less processing and greater control over data manipulation through functional programming constructs. RDD vs DataFrame differences also highlight trade-offs between flexibility and optimization. The underlying Directed Acyclic Graph (DAG) facilitates task execution and efficient Apache Spark architecture management.
Exploring RDD in Apache Spark Architecture
Every Spark application consists of a Driver program, which runs the primary function and is responsible for parallel operations on the cluster. The primary abstraction in Apache Spark is the RDD, which Spark uses for efficient MapReduce operations. A Resilient Distributed Dataset (RDD) is the fundamental data structure in Spark. They are immutable distributed collections of objects of any type and, as the name suggests, resilient (fault-tolerant) records of unstructured data that reside across multiple nodes.
Each Resilient Distributed Dataset (RDD) in Spark is divided into logical partitions across the cluster, enabling parallel operations on different cluster nodes. RDDs can be created through deterministic operations on data stored in stable storage or from an existing Scala collection or an external file in HDFS (or any other supported file system). Users can opt to persist an RDD in memory, allowing it to be reused multiple times efficiently. It also has the ability to recover automatically from faults in the system.
Key Features and Benefits of RDD in Spark
The key features of the resilient distributed dataset are:
Lazy Evaluation
All transformations in Apache Spark are lazy, meaning they don't compute the results immediately when the transformation is declared. Instead, they track the transformation tasks using the concept of Directed Acyclic Graph (DAG). Spark computes these transformations only when an action requires a result for the driver program.
In-Memory Computation
Spark uses in-memory computation to speed up processing time by keeping data in RAM (random access memory) rather than slower disk drives. This helps reduce memory costs and allows for more efficient pattern detection and large-scale data analysis. The cache() and persist() methods are key to this feature.
Fault Tolerance
RDDs are fault-tolerant because they can track data lineage, enabling automatic rebuilding of lost data in case of failure. To ensure fault tolerance, the data is replicated across various Spark Executors on worker nodes in the cluster.
Immutability
RDDs are immutable, making it easy to share data safely across multiple processes. Immutability prevents potential issues caused by concurrent updates from different threads. RDDs are not only immutable but also deterministic functions of their inputs, allowing for the recreation of data at any given instance. They can be thought of as a collection of data or a recipe for building new data from existing data.
Partitioning
Spark automatically partitions RDDs across multiple nodes when dealing with large volumes of data that cannot fit into a single node. Key points about RDD partitioning:
-
Each node in the Spark cluster contains one or more partitions.
-
Partitions do not span multiple machines.
-
The number of partitions in Spark is configurable and should be chosen efficiently.
-
By increasing the number of executors in the cluster, parallelism can be enhanced in the system.
Location Setup capability
RDDs in Spark can define placement preferences for compute partitions. Placement preference refers to the specification of the location where partitions should be placed. The DAG (Directed Acyclic Graph) comes into play here, ensuring that partitions are placed in a way that tasks are executed close to the data they need. This approach increases the speed of computation by minimizing data movement across the cluster.
A product of Apache Software Foundation, which is in an open-source unified programming model and is used to define and execute data processing pipelines. Click to explore about our, Apache Beam Architecture and Processing
Comparing RDD, Dataset, and DataFrame in Spark
In Apache Spark, understanding the distinctions between RDDs, Datasets, and DataFrames is crucial for optimizing performance and choosing the right abstraction for your use case. The following table provides a clear comparison of these three components, highlighting their unique features and benefits.
Feature |
RDD |
Dataset |
DataFrame |
Definition |
Fundamental data structure of Apache Spark. Immutable collections of objects across a cluster |
Strongly-typed, object-oriented data structure mapped to a relational schema |
Dataset organized into named columns, similar to a relational table |
Immutability |
Immutable (read-only) |
Immutable |
Immutable |
Type Safety |
No type safety (untyped) |
Strongly typed (type-safe) |
Not strongly typed (untyped) |
APIs |
RDD API |
Dataset API, extension of DataFrame API |
DataFrame API |
Language Support |
Supports Scala, Java, and Python |
Supports Scala, Java, and Python |
Supports Scala, Java, and Python |
Memory Management |
Requires manual management of memory |
Managed by Spark, optimized execution plans |
Provides memory management and optimized execution plans |
Fault Tolerance |
Fault-tolerant through lineage information |
Fault-tolerant, with support for persistence |
Fault-tolerant, with support for persistence |
Serialization |
Not serializable |
Serializable and queryable |
Serializable and queryable |
Performance |
Suitable for low-level transformations and fine-grained control |
Provides optimizations over RDDs via Catalyst optimizer |
Optimized for structured data with in-memory computations and query planning |
An open-source web framework that is used for building applications on AWS, Microsoft Azure, Kubernetes, etc. Click to explore about our, Serverless Solutions and Architecture for Big Data
Different Methods to Generate RDDs in Spark
Three ways to create an RDD in Apache Spark are listed below:Parallelizing Collection (Parallelized)
We take an already existing collection in the program and pass it to the SparkContext’s parallelize() method. This is a straightforward method for quickly creating RDDs in Spark-shell and performing operations on them. However, this method is rarely used as it requires the entire dataset to reside on one machine.
Referencing External Dataset
In Apache Spark, a Resilient Distributed Dataset (RDD) can be formed from any data source supported by Hadoop, including local file systems, HDFS, HBase, Cassandra, etc. Here, data is loaded from an external dataset. We can use SparkContext’s textFile method to create a text file by providing the URL of the file and reading it as a collection of lines. The URL can be a local path on the machine itself.
Creating RDD from an existing
Transformation mutates one RDD into another, and this is the way to create a new RDD from an existing one. This creates a key distinction between Apache Spark and Hadoop MapReduce. The conversion works like a function that takes an RDD and produces one. The input-resilient distributed dataset does not change, and since RDDs are immutable, it generates varying RDDs by applying operations.
Operation on RDD
There are two operations on RDDs in Spark: Transformations and Actions. A Transformation is a function that produces a new Resilient Distributed Dataset from an existing one. It takes an RDD as input and generates one or more RDDs as output. Every time a transformation is applied, a new RDD is created. Since RDDs are immutable, the input RDDs cannot be changed. Key points include:
-
No Change to the cluster.
-
Produces a Directed Acyclic Graph (DAG) that keeps track of which RDDs were created and when in the lifecycle.
-
Example: map(func), filter(func), Reduce(func), intersection(dataset), distinct(), groupByKey(), union(dataset), mapPartitions(fun), flatMap().
Presto queries data including Hive, Cassandra, relational databases, separating computation from storage performing independent scaling. Click to explore about our, Large Data Processing with Presto
Types of Transformations in Apache Spark RDD
-
Narrow Transformations: In this type, all the elements required to compute the records in a single partition live within that single partition. Here, a limited subset of partitions is used to calculate the result. Narrow transformations are the result of operations like map() and filter().
-
Wide Transformations: In wide transformations, the elements required to compute the records in a partition may reside in many of the partitions of the parent RDD. These transformations involve operations like groupByKey() and reduceByKey().
Spark Actions
Transformations in Apache Spark create new RDDs from existing ones, but to work on the actual dataset, we perform action operations. Actions do not produce new Resilient Distributed Datasets; instead, they return non-RDD values, which are stored on the Spark Driver or in an external storage system. This brings laziness to the processing.
-
Actions are responsible for sending data from Spark Executors to the Driver, where the Executors execute tasks, and the Driver is a JVM process that manages the workers and task execution.
-
Some Examples include : count(), collect(), take(n), top(), count value(), reduce(), fold(), aggregate(), foreach().
The Flow in the Apache Spark Architecture
When you enter code in the Spark Console, Apache Spark creates a graph representing the operations to be performed.
- When an action is called on Spark, the system submits the Directed Acyclic Graph (DAG) to the DAG Scheduler.
- The DAG Scheduler divides the operations into stages of tasks, which are then passed on to the Task Scheduler.
- The Task Scheduler launches the tasks through the Cluster Manager, which manages the execution of tasks across the cluster.
Limitations and Challenges with Apache Spark RDD
No Automatic Optimization
In Apache Spark, there is no automatic input optimization option. It cannot utilize advanced optimizers like the Catalyst optimizer and Tungsten execution engine. As a result, RDD optimization must be done manually. However, this limitation is overcome in the Dataset and DataFrame concepts, where both use the Catalyst optimizer to generate optimized logical and physical query plans, providing better space and speed efficiency.
No Static and Runtime Type Safety
Apache Spark does not provide static or runtime-type safety, making it difficult for users to detect errors at runtime. In contrast, Dataset provides compile-time type safety, allowing for the detection of errors during the compilation phase, which enhances the safety of the code.
The Problem of Overflow
Performance degrades when there isn't enough memory to store data in memory or on disk. In such cases, partitions that overflow from RAM may be stored on disk, which can impact performance. To overcome this issue, increasing the RAM and disk size is necessary.
Overhead of Serialization and Garbage Collection (Performance Limitation)
Apache Spark works with in-memory objects, which incurs the overhead of garbage collection and Java serialization, which can become expensive as the data grows. To mitigate this, we can use more efficient data structures with fewer objects or persistent objects in their serialized form.
No Schema View of Data
RDDs in Apache Spark have difficulty handling structured data because they do not provide a schema view. Unlike Dataset and DataFrame, which offer a schema view and organize data into named columns, RDDs lack such a provision, making them less suitable for handling structured data efficiently.
A technique that is used to convert the raw data into a clean data set before the execution of the Iterative Analysis. Click to explore about our, Data Processing in ML
Final Insights on Apache Spark RDD Performance
Hadoop MapReduce had several shortcomings that hindered performance and flexibility. To address these limitations, Spark RDD was introduced, offering in-memory processing, immutability, and other functionalities that provided users with a more efficient solution. However, RDDs also had their own limitations, restricting Apache Spark from being fully versatile. As a result, the concepts of DataFrames and Datasets were developed, further enhancing Spark's capabilities and addressing these constraints.
Recommended Next Steps for Spark Implementation
Connect with our experts to explore implementing advanced AI systems using RDDs in Apache Spark. Learn how industries leverage Agentic Workflows and Decision Intelligence to become decision-centric. RDDs enable AI to automate and optimize IT support and operations, enhancing efficiency and responsiveness.