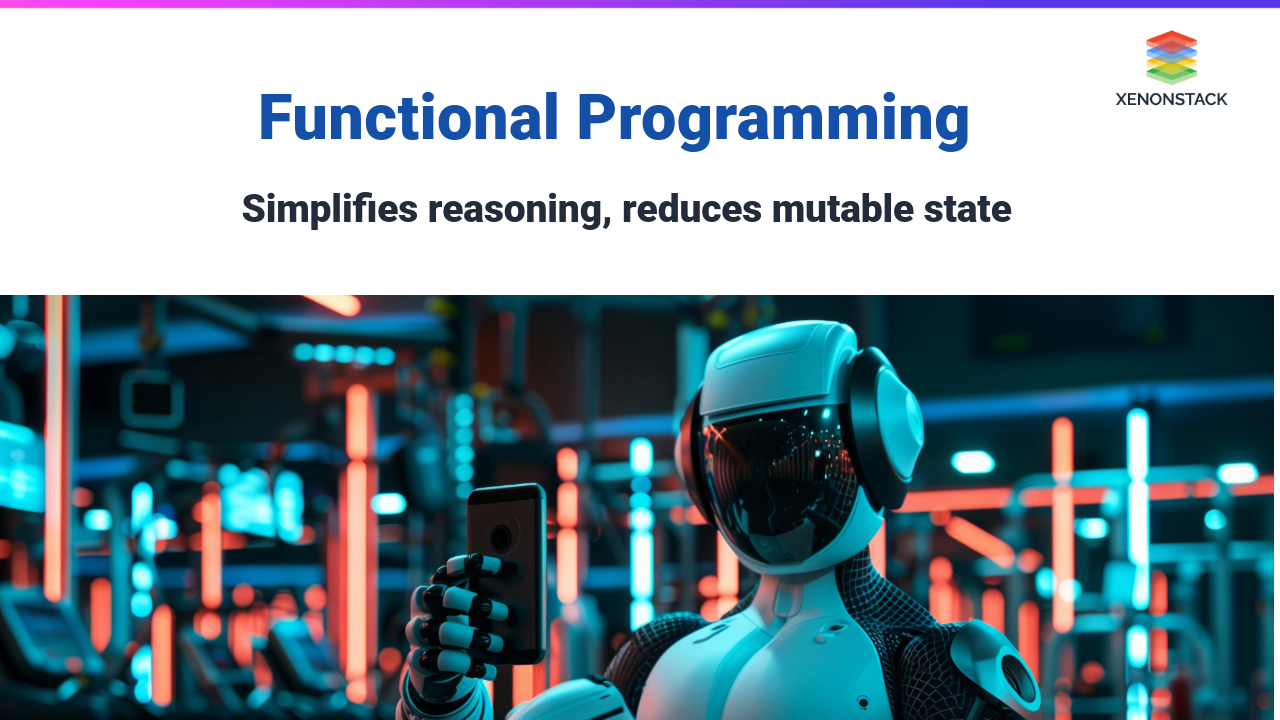
Introduction to functional programming languages
Functional programming (FP) is a powerful programming paradigm that emphasizes using pure functions and immutable values to create robust and maintainable applications. Unlike traditional imperative programming, which focuses on changing state and mutable data, functional programming treats computation as evaluating mathematical functions. This approach brings several benefits, making it increasingly popular in modern software development.
Key Principles of Functional Programming
Pure Functions
-
Always produce the same output for the same input
-
No side effects, which improves predictability
-
Simplifies debugging and testing by removing external influences
Immutability
-
Once a value is assigned, it cannot be changed
-
Reduces bugs related to mutable states
-
Enhances code clarity and maintainability
Core Concepts of Functional Programming Languages
Functional programming languages also introduce concepts like higher-order functions, which can accept other functions as arguments or return them as results, facilitating more abstract and reusable code. Function composition allows developers to build complex operations by combining simpler functions, leading to more modular and maintainable codebases.
As we explore the world of functional programming languages, we’ll uncover their key features, advantages, and popular languages that embody this paradigm. By understanding and applying functional programming principles, developers can create easier applications to debug, test, and scale, ultimately contributing to more efficient software development practices.
Functional programming has been a significant paradigm shift in the software world over the past 10 years. Source: Reducing Maintenance Costs With Functional Programming, Forbes
Here, the function should be Parametric. Everything happens within the function, and any outside expression does not alter it. This is crucial for Parallelism and Concurrency. Learn more about Functional Programming in Stream Analytics here.
What Defines a Functional Programming Language
For a language to be known as a functional programming language, it must follow the following methodologies:
Pure Functions
These functions help achieve a safe way of programming. Functional programming is all about pure functions. These functions only work on their input parameters, thus, limiting the scope of errors. Pure functions can be lazy. You can know about what the function implements by just looking at the signature of the pure functions. A few things that one needs to keep in mind while implementing a pure function are:
-
The pure function must take at least one parameter.
-
Pure functions never change the output, given that the input is kept the same too. This helps predict the behavior to some extent.
-
Pure functions do not have side effects. Thus, debugging is quite easy.
Immutability
In Functional Programming, a variable is immutable and cannot change its value once assigned. Immutability simplifies the code and enhances safety by avoiding side effects. It is essential when handling data to ensure that values remain consistent throughout the program.
Refactoring
Refactoring helps generalize functions so they can be reused in multiple places. This reduces the need for boilerplate code and makes the codebase more maintainable and modular.
Higher-Order Functions
In functional programming, functions are first-class citizens, treated like any other value (e.g., a variable). Higher-order functions either accept other functions as arguments, return functions, or do both, enabling more abstract, reusable, and modular code.
Functional Composition
In functional programming, function composition involves combining simpler functions to build more complex operations. This promotes reusability, as smaller, focused functions can be composed into more elaborate programs.
Currying
Currying transforms a function that expects multiple arguments into a series of functions, each accepting a single argument. This creates a chain of functions, allowing more flexible and modular code.
Referential Transparency
Referential transparency means that a function’s output depends solely on its input parameters. As long as the input remains the same, the output will always be identical, making the function predictable and easier to reason about.
Benefits of Embracing Functional Programming
Functional Programming (FP) offers several key advantages that make it an appealing choice for developers, particularly when building large, complex, and maintainable software systems.
-
Pure Functions: In FP, pure functions are at the core. These functions consistently return the same output for the same input, with no side effects, ensuring referential transparency. This makes the code more predictable and easier to reason about, resulting in fewer bugs and simplifying the debugging process.
-
Immutability: One of the significant advantages of functional programming is its emphasis on immutability. Once a value is assigned, it cannot be modified, which reduces the complexity of state management and eliminates the possibility of bugs arising from mutable data. Immutability ensures more reliable software with a clearer flow of data.
-
Higher-Order Functions: Higher-order functions (HOFs) allow functions to be passed as arguments or returned as values, enabling more modular and flexible code. These abstractions simplify complex problems, as functions can be composed or combined to perform complex tasks.
-
Declarative Programming: Unlike imperative programming, functional programming promotes a declarative approach. This means developers focus on "what" to do rather than "how" to do it, which leads to code readability and improved maintainability.
-
Recursion: In place of traditional loops, recursion is often used in FP to solve repetitive tasks. Recursion makes the code more elegant and enables the use of higher-order functions to handle repetitive logic in a clean, functional manner.
-
Concurrency and Parallelism: Functional programming languages handle concurrency and parallelism more efficiently. The absence of mutable states makes it easier to reason about multiple threads working on different parts of a program, improving performance without introducing data corruption.
Functional Programming vs. Object-Oriented Programming
The debate between functional programming and OOP has been long-standing, with each paradigm offering unique strengths. While object-oriented programming (OOP) is based on objects and classes used to model real-world entities, functional programming focuses on the evaluation of functions and avoids mutable states.
Aspect |
Functional Programming |
Object-Oriented Programming (OOP) |
State Management |
Avoids mutable state by using immutability, simplifying reasoning, and reducing bugs |
Focuses on managing the state of objects and their interactions, which can lead to complex and error-prone code |
Side Effects |
Eliminates side effects through pure functions, ensuring output depends solely on input, making it predictable |
Objects can modify their internal state, introducing side effects and potentially causing unpredictable behaviors |
Abstractions and Composition |
Uses function composition, higher-order functions, and currying to create modular, reusable code |
Relies on classes, inheritance, and polymorphism for abstractions, which can lead to more complex and less modular code |
Concurrency |
Better suited for concurrency and parallelism due to the absence of mutable state, reducing thread safety issues |
Concurrency is challenging due to mutable shared states, making thread safety and resource management more difficult |
Code Readability and Maintainability |
Results in more concise, modular, and readable code, especially with features like first-class functions, closure, and lazy evaluation |
Often leads to more verbose and tightly coupled code, which can be harder to maintain and test |
Real-World Applications of Functional Programming
Functional Programming is widely used across various domains and offers significant advantages in certain types of applications. Here are a few examples:
-
Concurrency and Parallelism: FP's emphasis on immutability and pure functions makes it ideal for applications requiring high levels of concurrency or parallelism, such as real-time data processing, scientific computing, and big data systems. FP reduces the risk of race conditions and improves performance across distributed systems.
-
Web Development: Many modern web development frameworks and libraries (e.g., Functional Libraries in JavaScript) leverage Functional Programming principles to build scalable and maintainable applications. JavaScript, being a multi-paradigm language, allows developers to utilize functional patterns to enhance performance, manage state effectively, and ensure consistency across the application.
-
Domain-Specific Languages (DSLs): Functional programming is frequently used to design Domain-Specific Languages (DSLs) due to its ability to create abstractions and handle complex transformations elegantly. For instance, Monads are a popular concept in FP, used to handle side effects and manage computations in a controlled manner.
-
Data Transformation: FP shines in data-driven applications that involve complex transformations. Through techniques like function composition, partial application, and recursion, developers can manipulate large datasets in a clear and efficient manner. These features make FP an excellent choice for building ETL (Extract, Transform, Load) pipelines.
-
Financial and Security Systems: Pure functions and referential transparency make FP an ideal paradigm for developing systems in industries like finance and security. These features ensure consistency and predictability, which are critical for the correctness of financial calculations or cryptographic algorithms.
Key Takeaways from Functional Programming
Keeping these principles in mind while creating code using the functional programming paradigm will result in an application that is easier to understand, simpler to debug, reusable, free of side effects, and capable of being used in parallelism and concurrency. By adhering to functional programming principles like pure functions, immutability, and referential transparency, developers can build more predictable and maintainable software.
Moving Forward with Functional Programming
Talk to our experts about implementing Functional Programming in complex systems, how industries and departments leverage pure functions, immutability, and recursion to optimize workflows and decision-making. Functional Programming enhances efficiency and scalability by automating IT operations and improving responsiveness, enabling organizations to streamline processes and manage large-scale systems effectively.