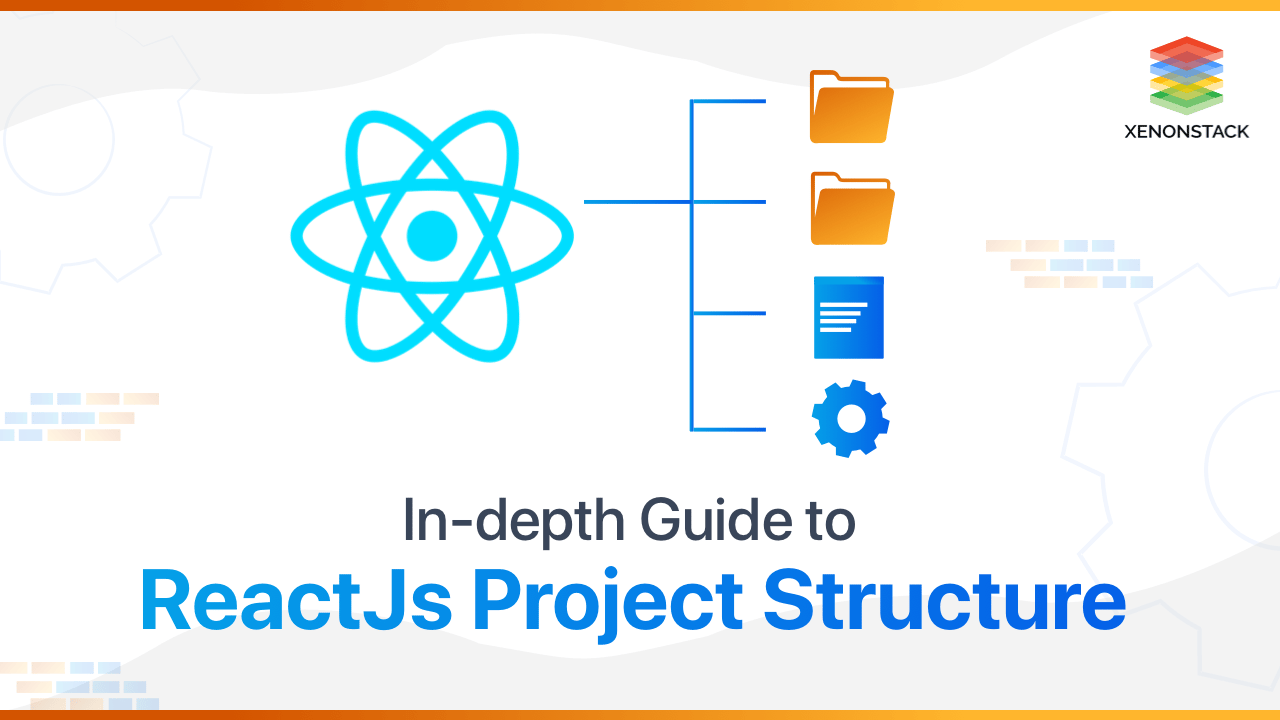
Overview of Reactjs Project Structure
ReactJS, a popular JavaScript library for building user interfaces, boasts a flexible and component-based architecture that enables the creation of dynamic and interactive web applications. Understanding the ReactJS project structure is essential for developers to ensure maintainability, scalability, and effective collaboration within teams. This introduction will outline the key components of a typical ReactJS project structure, highlighting best practices for organizing files and directories to improve clarity and efficiency in development.
Understanding React and Its Core Features
React is a JavaScript library created by Facebook, serving as a User Interface (UI) library and a tool for building UI components. To ensure a maintainable and scalable project, it's important to follow best practices when structuring your app. Often, when using create-react-app, the app is generated with no build configuration. In this blog, we will build our own boilerplate and examine the ideal folder structure and React project structure that most of the community prefers, focusing on file organization strategies, scalability in React apps, and React best practices.
Exploring the Architecture of React Applications
React is primarily a UI library and does not enforce a specific design pattern for building complex applications, giving developers flexibility in choosing their own approach. The React community advocates for using the Flux pattern for better structure and maintainability. This pattern helps in managing the data flow within a React application.
Key React Concepts
-
Higher-Order Components: Used to enhance component functionality by wrapping them with additional behavior.
-
Context: Enables passing data through the component tree without manually passing props at every level, making state management more efficient.
-
Render Props: A pattern that allows components to share code by passing a function as a prop.
-
Refs: Provide a way to access and interact with DOM elements or React components directly.
These core concepts and design patterns are essential for building scalable and maintainable React applications, ensuring cleaner, more efficient, and reusable code.
A process which is used for increasing the quality of a software or a product and for improving it by identifying defects, problems, and errors. Click to explore about JavaScript and ReactJS Unit Testing
Guide to Structuring Your React Project
When starting a React project, one of the key decisions is how to organize your files and directories. While there is no one-size-fits-all solution, it’s crucial to have a clear and logical structure in place. Here are some key points to consider:
No One-Size-Fits-All Architecture
There is no universal "best project architecture" that fits every project or coding style. Each project might require a different approach based on its complexity, team, and goals.
Importance of Project Structure
While React doesn’t enforce a specific structure, it’s essential to have a well-organized project. The lack of a defined structure allows flexibility but also requires developers to create a design that suits their needs.
Flexibility to Choose
React gives the freedom to decide how to structure your project, which can be both an advantage and a challenge. You can choose to adopt an opinionated structure or create one from scratch based on your project requirements.
Adopting Best Practices
While there’s no single right way to structure a React project, adopting some best practices will help improve maintainability, scalability, and collaboration. You can incorporate elements like feature folders, modular design, and component-based architecture for better clarity.
Customized Approach
The structure you choose should be tailored to your project’s specific needs. The following discussion offers a structured approach to organizing your React project, but you can use some or all of it depending on your project's requirements.
Fig 1: Structure for Setting React Project
Steps to Set Up Your React Project
First, let’s set up the development environment:
-
Code Editor: Use VS Code with various extensions to enhance your development experience.
-
Browser: Google Chrome is a great choice, offering multiple developer-friendly tools for debugging.
-
Debugging Tools: React Developer Tools and Redux DevTools are essential for efficiently debugging and inspecting your React and Redux state.
Now we can use CLI to create a new project.
Reactjs that are created using ‘create-react-app' give some default code. Let’s delete almost everything, like logos, images, styling, etc., and keep the skeleton application. Now, we can start setting up the ideal folder structure for our Reactjs project.
Understanding the Folder Structure in React
The folder structure looks like this.
-
Assets Folder
-
Layouts Folder
-
Components Folder
-
Pages Folder
-
Middleware Folder
-
Routes Folder
-
Config Folder
-
Services Folder
-
Utils Folder
Assets Folder
As the name suggests, this folder contains the assets for our React project. It includes images and styling files. We can store our global styles here, centralizing the project and storing page-based or component-based styles as needed. However, styling can also be stored within the pages folder or components folder, depending on the developer's comfort.
Layouts Folder
This folder contains layouts available to the entire project, such as the header, footer, sidebar, etc. We can store the header, footer, or sidebar code here and call it wherever necessary, promoting reusable components.
Components Folder
Components are the building blocks of any React project. This folder holds UI components like buttons, modals, inputs, loaders, etc., which can be reused throughout the application. Each component should include a test file for unit testing, as these components are widely used across the project.
Pages Folder
The files in the pages folder represent the routes of the React application. Each file in this folder corresponds to a specific route. A page can also have subfolders for organization. Each page has its own state and is typically used to trigger asynchronous operations. Pages often consist of various components grouped together.
Middleware Folder
This folder contains middleware for handling side effects within the application. It is especially useful when integrating Redux or state management solutions. Here, custom middleware for handling specific tasks, like logging or error handling, can be stored.
Routes Folder
This folder manages all the application's routes, including private, protected, and public routes. It can also handle nested or sub-routes, helping in organizing routing in React.
Config Folder
The config folder stores configuration files, such as environment variables, in a file like config.js. This folder helps set up multi-environment configurations for the application.
Services Folder
This folder is necessary when using Redux or other state management solutions. It contains three subfolders: actions, reducers, and constants. These folders manage the application's state and actions, and their organization follows the page folder structure for clarity.
Utils Folder
The utils folder holds utility functions that are used across the project. It should contain general-purpose JavaScript functions and objects, like dropdown options, regex conditions, data formatting functions, etc. These utilities promote modular design and code reusability.
Key Pitfalls to Avoid in React Projects
Overcomplicating Folder Structure: Avoid creating an overly complex React folder structure. Aim for simplicity and clarity to enhance development efficiency. Not Using Reusable Components: Failing to create reusable components can lead to repetitive code, making it harder to scale and maintain the app. Neglecting Performance Optimization: Don't ignore code splitting and lazy loading. These strategies improve app performance by reducing initial load times. Skipping Linting and Type Checking: Linting and type checking with tools like ESLint and TypeScript prevent common errors and maintain code consistency. Ignoring Routing in React: Use React Router effectively to handle routing in React and avoid overly complex routing logic to improve navigation. Lack of Error Handling: Proper debugging and error handling mechanisms are essential for identifying and resolving issues in production. Not Modularizing Code: Keep your codebase modular by grouping related functionality together to enhance scalability in React apps and ease maintenance.
Key Best Practices for React Project Organization
When it comes to Reactjs development, organizing your Reactjs project structure is crucial for ensuring maintainability, scalability, and ease of collaboration. Here are some of the key React best practices to follow:
-
Component-Based Architecture: Break the UI into reusable components for easier maintenance and updates, such as buttons and input fields.
-
Feature-Focused Folders: Organize the project by feature, grouping components, actions, and reducers together for better scalability.
-
Pages Folder: The top-level components representing distinct views should be stored in the pages folder to keep the structure clean and organized.
-
Layouts Folder: Reusable layout components like the Header, Footer, and Sidebar should be stored in the layouts folder to ensure consistent design.
-
Assets Folder: Store static resources, such as images, icons, and fonts, in the assets folder for easy management.
-
Naming Conventions: Adopt PascalCase for components, camelCase for variables, and kebab-case for file names to ensure consistency.
-
State Management: Leverage Redux or the Context API to manage the global state with a dedicated folder for state logic.
-
Testing in React: Implement unit tests, integration tests, and snapshot testing using tools like Jest and React Testing Library to ensure proper functionality.
-
Code Splitting: Use code-splitting to improve performance by loading parts of the app only when needed, reducing initial load time.
-
CSS Organization: Organize styles with CSS Modules or styled-components to prevent global style conflicts.
Final Thoughts on Reactjs Project Structure
In conclusion, establishing an efficient project structure for React.js is essential for maintaining clarity, scalability, and ease of collaboration. A well-organized structure rooted in a component-based architecture promotes modularity by allowing developers to create reusable components that are easier to test, maintain, and navigate. As projects expand, a clear directory layout becomes increasingly important, allowing for straightforward management of files and components, whether through feature-based grouping or a separation of presentation and logic.
This separation of concerns helps isolate responsibilities and simplifies debugging and feature enhancements. Integrating tools like ESLint and Prettier early on maintains code quality and consistency, especially in team environments. Good documentation also helps new team members understand the codebase quickly. Lastly, being adaptable in your project organization ensures your structure evolves with the application, allowing it to face future challenges while maintaining a clean and efficient development process.
Actionable Next Steps for React Development
Talk to our experts about implementing an optimized Reactjs project structure. Learn how industries and departments use Agentic Workflows and Decision Intelligence to become decision-centric. Leverage React best practices to automate and streamline IT support and operations, enhancing efficiency and responsiveness.